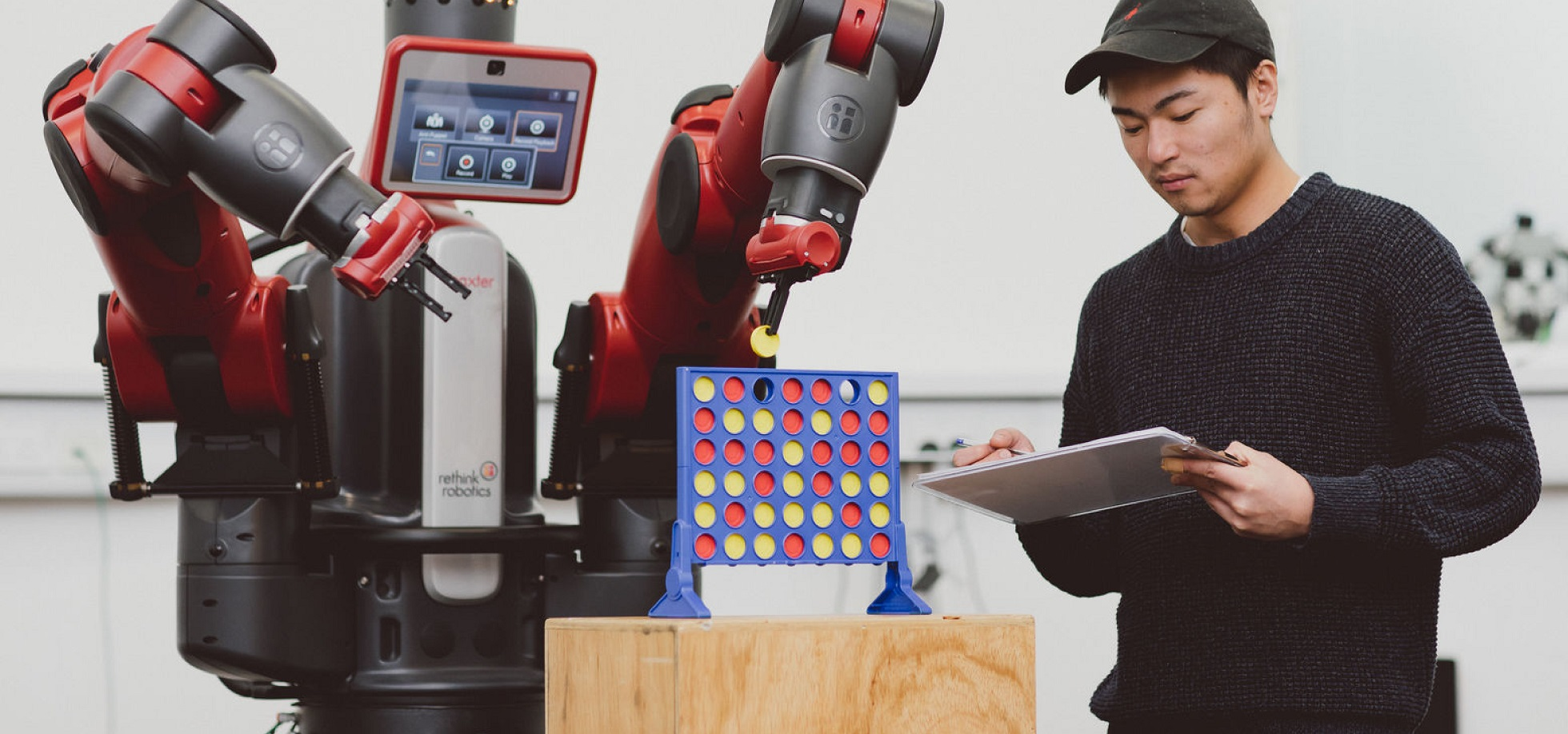
Module Description
The aim of this module is to provide an introduction to the C++ programming language. The contents covered by this module
include three parts: (1) basic concepts and features of C++ programming (e.g., operator overloading),
(2) C++ Standard Template Library, and (3) inheritance, function overriding and exceptions.
Learning Outcomes
After completing this module, students will be expected to be able to:
1. Explain the basic concepts and features of C++.
2. Describe the underlying memory model and explain the role of the execution stack and the heap.
3. Write object oriented programs that incorporate basic C++ features such as pointer, reference, inheritance, function overriding, operator overloading, exceptions, etc.
4. Make effective use of the C++ Standard Template Library.
Outline Syllabus
Overview
- paradigms: procedural + object-oriented vs purely object-oriented
- pointers and references
- C++ functions: call by value and call by reference
- class definition
- memory management
- the C++ Standard Template Library
A model of memory
- static and dynamic memory
- the execution stack: existence of local variables, parameter passing
- the heap: dynamic memory allocation
- memory management in C++
C++ language features
- pointers: declaring and using pointer variables, the operator *
- references: declaring and using reference variables, the operator &
- functions: call by value and call by reference, function overloading
- arrays: array identifiers, static and dynamic arrays, arrays as parameters, arrays as results
- class definition: private and public members
- inheritance
- function overriding (polymorphism)
- operator overloading
- constructors, destructors, copy constructors and the assignment operator